.:: Spring Mass Mechanics Simulator ::.
Spring Mass Simulator
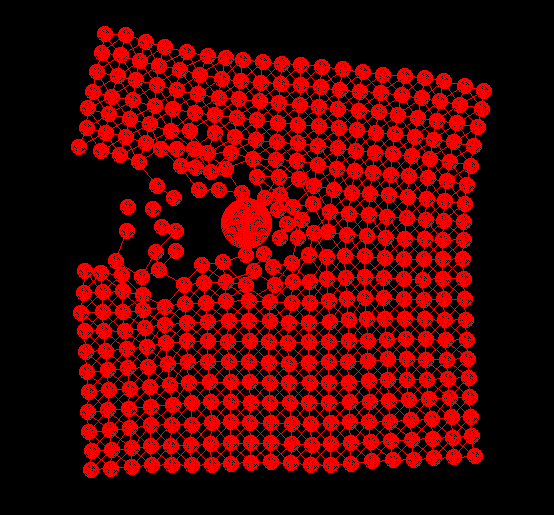
This is a spring mass system. In theory, you can simulated anything in classical physics using a spring mass system.
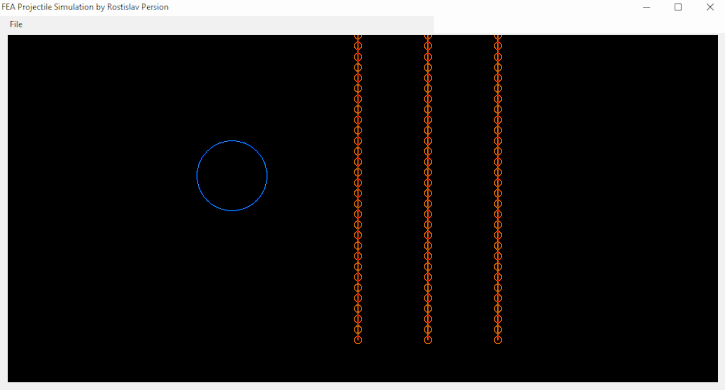
DOWNLOAD SPRING MASS LIBRARY >>
SOFTWARE WRITTEN IN C#
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 | using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Persion.SpringMass { public class Mass { public bool Fixed = false; public double x = 0; public double y = 0; public double vx = 0; public double vy = 0; public double ax = 0; public double ay = 0; public double fx = 0; public double fy = 0; public double mass = 10; public double diameter = 20; public Queue<Spring> Springs = new Queue<Spring>(); public Queue<Collider> Colliders = new Queue<Collider>(); public void ConnectTo(Mass mass2, double k, double MaxLengthFactor) { Spring newSpring = new Spring(); newSpring.k = k; newSpring.node = mass2; newSpring.length = Mass.Distance(this, mass2); newSpring.maxlength = MaxLengthFactor * newSpring.length; Springs.Enqueue(newSpring); } public void CollideWith(Mass mass2, double k) { Collider newCollider = new Collider(); newCollider.k = k; newCollider.node = mass2; newCollider.length = (this.diameter / 2) + (mass2.diameter / 2); Colliders.Enqueue(newCollider); } public void Move(double dt, double gravity) { if (!Fixed) { ax = fx / mass; ay = (fy / mass) + gravity; vx += (ax * dt); vy += (ay * dt); x += (vx * dt); y += (vy * dt); } fx = 0; fy = 0; } public void Forces() { foreach (Spring sp in Springs) { if (Mass.Distance(this, sp.node) >= sp.maxlength) { sp.broken = true; } if (!sp.broken) { this.fx += (((Mass.Distance(this, sp.node) - sp.length) * sp.k) / sp.length) * (sp.node.x - this.x); this.fy += (((Mass.Distance(this, sp.node) - sp.length) * sp.k) / sp.length) * (sp.node.y - this.y); sp.node.fx += (((Mass.Distance(this, sp.node) - sp.length) * sp.k) / sp.length) * (this.x - sp.node.x); sp.node.fy += (((Mass.Distance(this, sp.node) - sp.length) * sp.k) / sp.length) * (this.y - sp.node.y); } } } public void Collide() { foreach (Collider cl in Colliders) { if (Mass.Distance(this, cl.node) <= ((this.diameter / 2) + (cl.node.diameter / 2))) { this.fx += (((Mass.Distance(this, cl.node) - cl.length) * cl.k) / cl.length) * (cl.node.x - this.x); this.fy += (((Mass.Distance(this, cl.node) - cl.length) * cl.k) / cl.length) * (cl.node.y - this.y); cl.node.fx += (((Mass.Distance(this, cl.node) - cl.length) * cl.k) / cl.length) * (this.x - cl.node.x); cl.node.fy += (((Mass.Distance(this, cl.node) - cl.length) * cl.k) / cl.length) * (this.y - cl.node.y); } } } public static double Distance(Mass mass1, Mass mass2) { return Math.Sqrt(Math.Pow(mass1.x - mass2.x, 2) + Math.Pow(mass1.y - mass2.y, 2)); } } public class Spring { public double k = 1; public double length = 1; public double maxlength = 2; public bool broken = false; public Mass node; } public class Collider { public double k = 1; public double length = 1; public Mass node; } } |