.:: Smart MD5 decoder ::.
Reverse MD5 hashes with custom search settings
Crack MD5 hashes, by choosing what to look for, including a list of possible words that might be in the hash.
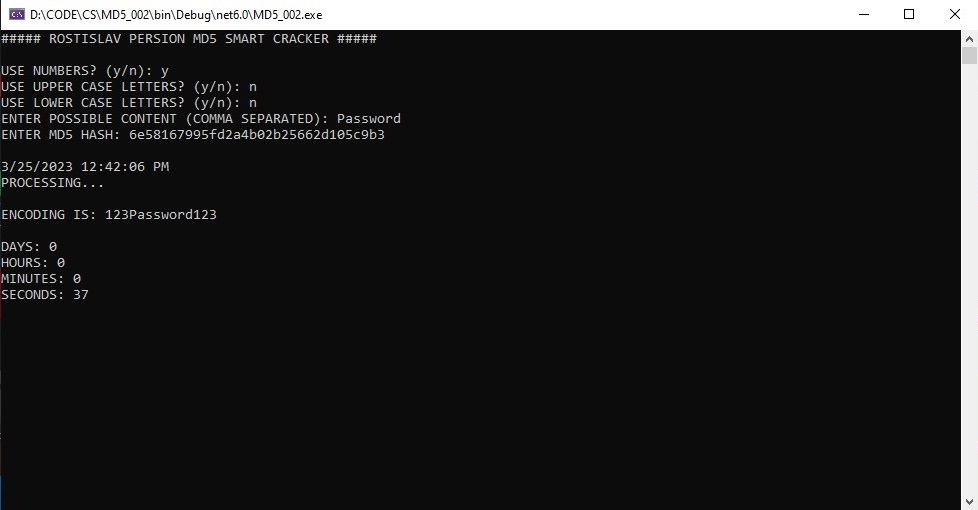
Here is a PNG file with an encoded message...
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 | using System.Text; using System.Security.Cryptography; Console.WriteLine("##### ROSTISLAV PERSION MD5 SMART CRACKER #####"); Console.WriteLine(); // SETTINGS AND BUILD LEX string lex1 = ""; string res1 = ""; Console.Write("USE NUMBERS? (y/n): "); res1 = Console.ReadLine().ToUpper().Trim(); if (res1 == "Y") { lex1 += "0,1,2,3,4,5,6,7,8,9,"; } Console.Write("USE UPPER CASE LETTERS? (y/n): "); res1 = Console.ReadLine().ToUpper().Trim(); if (res1 == "Y") { lex1 += "A,B,C,D,E,F,G,H,I,J,K,L,M,N,O,P,Q,R,S,T,U,V,W,X,Y,Z,"; } Console.Write("USE LOWER CASE LETTERS? (y/n): "); res1 = Console.ReadLine().ToUpper().Trim(); if (res1 == "Y") { lex1 += "a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z,"; } lex1 = lex1.TrimEnd(','); // input possible content Console.Write("ENTER POSSIBLE CONTENT (COMMA SEPARATED): "); string content = Console.ReadLine(); content = " ," + content.Replace(" ", string.Empty) + "," + lex1; string[] lex2 = content.Split(','); // enter md5 hash Console.Write("ENTER MD5 HASH: "); string md5hash = Console.ReadLine(); // timing DateTime start_time = new DateTime(); start_time = DateTime.Now; Console.WriteLine(); Console.WriteLine(DateTime.Now); Console.WriteLine("PROCESSING..."); // MD5 INIT MD5 md5Hash = MD5.Create(); byte[] data; StringBuilder sBuilder = new StringBuilder(); // itteration string try_string = ""; for (int a = 0; a < lex2.Length; a++) { for (int b = 0; b < lex2.Length; b++) { for (int c = 0; c < lex2.Length; c++) { for (int d = 0; d < lex2.Length; d++) { for (int e = 0; e < lex2.Length; e++) { for (int f = 0; f < lex2.Length; f++) { for (int g = 0; g < lex2.Length; g++) { for (int h = 0; h < lex2.Length; h++) { // create an md5 attempt try_string = (lex2[a] + lex2[b] + lex2[c] + lex2[d] + lex2[e] + lex2[f] + lex2[g] + lex2[h]).Trim(); //debug stuff //Console.WriteLine(try_string); //Console.WriteLine(GetMd5Hash(try_string)); // MD5 INNER sBuilder.Clear(); data = md5Hash.ComputeHash(Encoding.UTF8.GetBytes(try_string)); for (int i = 0; i < data.Length; i++) { sBuilder.Append(data[i].ToString("x2")); } // check for match if (md5hash == sBuilder.ToString()) { // result Console.WriteLine(); Console.WriteLine("ENCODING IS: " + try_string); //timing DateTime end_time = DateTime.Now; TimeSpan diff_time = end_time - start_time; Console.WriteLine(); Console.WriteLine("DAYS: " + diff_time.Days); Console.WriteLine("HOURS: " + diff_time.Hours); Console.WriteLine("MINUTES: " + diff_time.Minutes); Console.WriteLine("SECONDS: " + diff_time.Seconds); Console.ReadLine(); return; } } } } } } } } } |