.:: Simple Shape Recognition ::.
Very simple shape recognition algorithm using C#
This is a very simple program that classifies simple shapes in a 2x2 matrix.
LINK TO PHP WEB VERSION 2x2 >>
LINK TO PHP WEB VERSION 3x3 >>
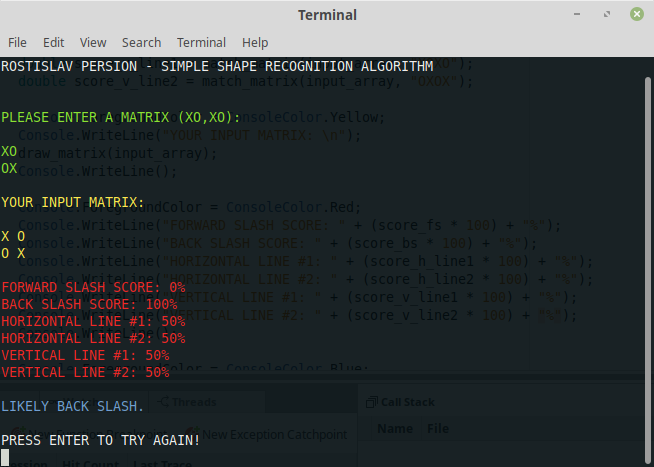
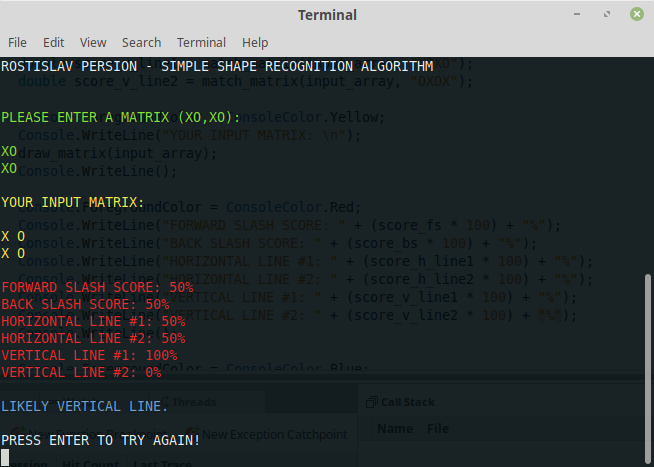
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | using System; namespace Neural_NetTest_001 { class MainClass { public static void draw_matrix(string array_in) { Console.Write(array_in.Substring(0, 1) + " "); Console.Write(array_in.Substring(1, 1) + "\n"); Console.Write(array_in.Substring(2, 1) + " "); Console.Write(array_in.Substring(3, 1) + "\n"); } public static double match_matrix(string array_in, string array_test) { int same = 0; int diff = 0; if (array_in.Substring(0, 1) == array_test.Substring(0, 1)) { same++; } else { diff++; } if (array_in.Substring(1, 1) == array_test.Substring(1, 1)) { same++; } else { diff++; } if (array_in.Substring(2, 1) == array_test.Substring(2, 1)) { same++; } else { diff++; } if (array_in.Substring(3, 1) == array_test.Substring(3, 1)) { same++; } else { diff++; } double score = (double)same / ((double)same + (double)diff); return score; } public static void Main(string[] args) { while (true) { Console.Clear(); Console.ForegroundColor = ConsoleColor.White; Console.WriteLine("ROSTISLAV PERSION - SIMPLE SHAPE RECOGNITION ALGORITHM\n\n"); Console.ForegroundColor = ConsoleColor.Green; Console.Write("PLEASE ENTER A MATRIX (XO,XO): \n\n"); string input_array = Console.ReadLine().Trim().ToUpper() + Console.ReadLine().Trim().ToUpper(); Console.WriteLine(); // score it double score_fs = match_matrix(input_array, "OXXO"); double score_bs = match_matrix(input_array, "XOOX"); double score_h_line1 = match_matrix(input_array, "XXOO"); double score_h_line2 = match_matrix(input_array, "OOXX"); double score_v_line1 = match_matrix(input_array, "XOXO"); double score_v_line2 = match_matrix(input_array, "OXOX"); Console.ForegroundColor = ConsoleColor.Yellow; Console.WriteLine("YOUR INPUT MATRIX: \n"); draw_matrix(input_array); Console.WriteLine(); Console.ForegroundColor = ConsoleColor.Red; Console.WriteLine("FORWARD SLASH SCORE: " + (score_fs * 100) + "%"); Console.WriteLine("BACK SLASH SCORE: " + (score_bs * 100) + "%"); Console.WriteLine("HORIZONTAL LINE #1: " + (score_h_line1 * 100) + "%"); Console.WriteLine("HORIZONTAL LINE #2: " + (score_h_line2 * 100) + "%"); Console.WriteLine("VERTICAL LINE #1: " + (score_v_line1 * 100) + "%"); Console.WriteLine("VERTICAL LINE #2: " + (score_v_line2 * 100) + "%"); Console.WriteLine(); Console.ForegroundColor = ConsoleColor.Blue; if(score_fs >= 0.75) { Console.WriteLine("LIKELY FORWARD SLASH."); } if (score_bs >= 0.75) { Console.WriteLine("LIKELY BACK SLASH."); } if ((score_h_line1 >= 0.75) || (score_h_line2 >= 0.75)) { Console.WriteLine("LIKELY HORIZONTAL LINE."); } if ((score_v_line1 >= 0.75) || (score_v_line2 >= 0.75)) { Console.WriteLine("LIKELY VERTICAL LINE."); } Console.ForegroundColor = ConsoleColor.White; Console.WriteLine(); Console.WriteLine("PRESS ENTER TO TRY AGAIN!"); Console.ReadLine(); } } } } |