.:: Spring Mass Fourier Transform ::.
Fourier Transform using Spring Mass System
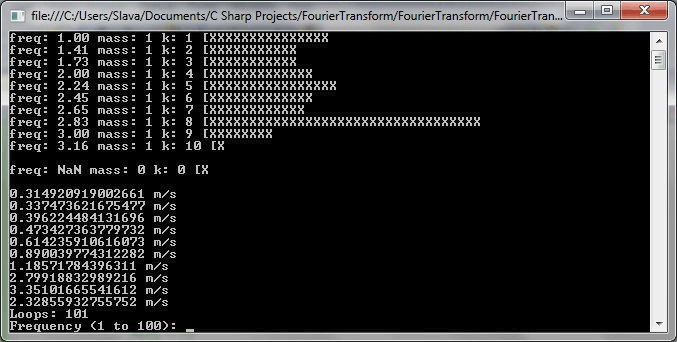
This is a fourier transform demo based on the concept of tuning forks and sping mass systems. A spring and a mass have a resonance frequency. By feeding an analog signal to a set of springs and masses you will see some of them resonate. By measuring their average velocity, you can determine the frequencies in a signal.
SOFTWARE WRITTEN IN C#
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 | using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading; namespace ConsoleApplication1 { class Program { static void Main(string[] args) { while (true) { int n = 10; double[] k = new double[] {1,8,15,22,29,36,43,50,57,64}; double[] m = new double[] {1,1,1,1,1,1,1,1,1,1}; double[] x = new double[n]; double[] vx = new double[n]; double[] ax = new double[n]; double dt = .1; double t = 0; double[] av = new double[n]; int avcounter = 0; for (int i = 0; i < n; i++) { x[i] = 0; vx[i] = 0; ax[i] = 0; } //clear average for (int i = 0; i < n; i++) { av[i] = 0; } //get sine wave frequency Console.Write("Frequency (1 to 100): "); double sinfreq = Convert.ToDouble(Console.ReadLine()); while (t < 10) { for (int i = 0; i < n; i++) { ax[i] = (forcefunction(t,sinfreq) + (-x[i] * k[i])) / m[i]; vx[i] += 2 * ax[i] * dt; x[i] += 2 * vx[i] * dt; } t += dt; //count averages avcounter++; for (int i = 0; i < n; i++) { av[i] += Math.Abs(vx[i]); } //draw Console.Clear(); DisplayMass(x[0], m[0], k[0]); DisplayMass(x[1], m[1], k[1]); DisplayMass(x[2], m[2], k[2]); DisplayMass(x[3], m[3], k[3]); DisplayMass(x[4], m[4], k[4]); DisplayMass(x[5], m[5], k[5]); DisplayMass(x[6], m[6], k[6]); DisplayMass(x[7], m[7], k[7]); DisplayMass(x[8], m[8], k[8]); DisplayMass(x[9], m[9], k[9]); Console.WriteLine(); DisplayMass(forcefunction(t,sinfreq), 0, 0); Thread.Sleep(50); } //count averages Console.WriteLine(); for (int i = 0; i < n; i++) { av[i] /= avcounter; Console.WriteLine(av[i] + " m/s"); } Console.WriteLine("Loops: " + avcounter.ToString()); } } public static double forcefunction(double t, double f) { return (Math.Sin(t / (2 * Math.PI) * f)); } public static void DisplayMass(double x,double m, double k) { string buff = "freq: " + String.Format("{0:0.00}", Math.Sqrt(k / m)) + " mass: " + m.ToString() + " k: " + k.ToString() + " ["; int num = (int)Math.Floor(10 * x)+10; for (int i = 0; i < num; i++) { buff += "X"; } buff += "X"; Console.WriteLine(buff); } } } |